API Quick Start
Step by step guide to using the API
This Quickstart will help you become more familiar with the Arcadia API by collecting statement and interval data as simply as possible. 🏁
Setting up the API
Below is a typical API workflow to collect all statement and interval data related to a credential:
- Request an access token to make API calls using your API keys
- Create a credential
- Fetch Statements found under the Credential
- Filter meters in preparation of turning on (or "activating" in Plug parlance) interval data
- Activate the interval data product for a particular meter
- Request interval data for a particular meter once it's collected
Let's jump into more detail for each step below.
1. Create an Access token
Grab your Client ID and Client Secret from the Dashboard's Configuration section. You'll be sending the ID and Secret to the OAuth endpoint to generate an Access Token which you can use in subsequent requests. Access Tokens are valid for one hour after which you'll need to request a new one.
curl --request POST \
--url https://api.arcadia.com/oauth2/token \
--header 'accept: application/json' \
--header 'content-type: application/x-www-form-urlencoded' \
--data grant_type=client_credentials \
--data client_id={INSERT_YOUR_CLIENT_ID} \
--data client_secret={INSERT_YOUR_CLIENT_SECRET}
{
"access_token": "abcd_123", // Copy your access token for future steps
"expires_in": 300,
"refresh_expires_in": 0,
"token_type": "Bearer",
"not-before-policy": 0,
"scope": "profile email"
}
2. Creating a credential
You can either submit credentials by using Connect or by submitting username and password via the API. Connect is our off-the-shelf user interface that enables end-users to select their utility provider and submit their login credentials.
Using Connect is typically faster and easier for getting up and running so that's what we'll use for this Quickstart. You can find your organization's specific Connect URL on the Connect Configuration page of the Dashboard.
Add a correlationId
to your Connect session. A correlationId
is simply a unique identifier you provide so that you can easily associate statements and intervals with your own customer identifier. It can be any set of characters like "api-quickstart"
. For the purpose of this Quickstart, the Dashboard offers a handy facility for adding your correlationId
to the Connect URL – Just type in an identifier and hit the "Copy Link" button.
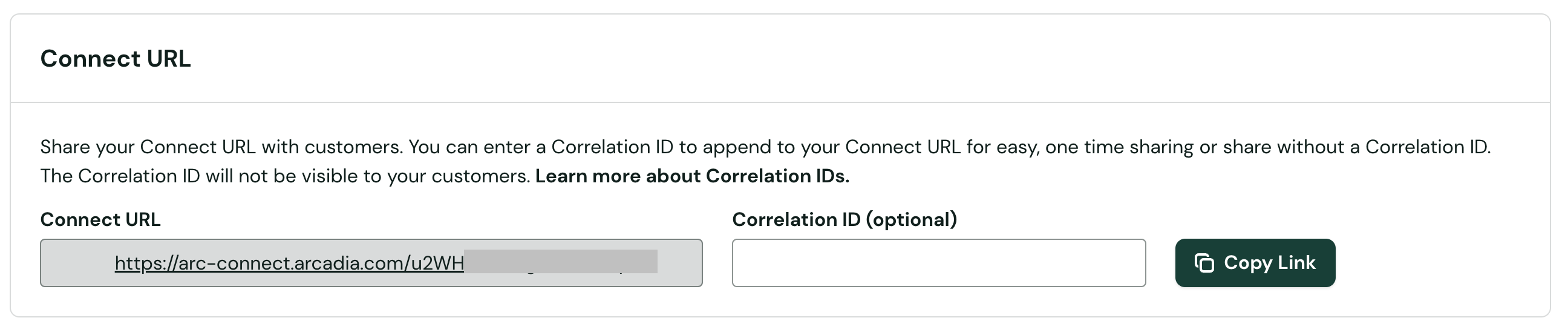
Paste the URL into your browser and complete the flow within Connect to select a provider and submit your login credentials to that provider.
3. Fetch Statements found under the Credential
Once the credentials have been successfully submitted, Arcadia will begin collecting data about each of their accounts, all of the statements at each account, and all the meters on the statements. This process can take anywhere from a few minutes to potentially hours if there are issues with the provider (like their infrastructure is down).
We recommend using Webhook Events for a production environment, but for the purpose of this Quickstart, we can poll the REST API to list the Statements associated with the correlationId
. You'll need two pieces of information from previous steps:
- The Access Token from Step 1
- The Correlation ID you used in Step 2
In the example below plug in your own accessToken
and correlationId
curl --request GET \
--url 'https://api.arcadia.com/plug/statements?search=correlationIds%3D%3D{correlationId}&page=0&size=20' \
--header 'Arcadia-Version: 2024-02-21' \
--header 'accept: application/json' \
--header 'authorization: Bearer {accessToken}'
It may take a few minutes, but eventually you'll start seeing Statements being returned. The response will take a format like:
{
"page": {
"number": 0,
"size": 0,
"totalElements": 0,
"totalPages": 0
},
"statements": [
{
"accountData": [
{
"accountId": "string",
"accountNumber": "string",
"amountDue": 0,
"dueDate": "2024-12-12",
"meterData": [
{
"amountDue": 0,
"currencyCode": "USD",
"meterId": "string",
"meterNumber": "string",
"meterReadDate": "2024-12-12",
"normalizedMeterNumber": "string",
"normalizedPointOfDeliveryNumber": "string",
"outstandingBalance": 0,
"pointOfDeliveryNumber": "string",
"previousReadDate": "2024-12-12",
"serviceType": "string",
"serviceTypeClassification": "CORE",
"totalCharges": 0,
"totalUsage": 0,
"totalUsageUnit": "string"
}
],
"normalizedAccountNumber": "string",
"outstandingBalance": 0,
"provider": {
"classification": "PUBLISHER",
"id": "string",
"name": "string",
"publisherProviderAccountId": "string"
},
"totalCharges": 0
}
],
"amountDue": 0,
"correlationIds": [
"string"
],
"createdAt": "2024-12-12T22:33:14.975Z",
"currencyCode": "USD",
"dataIngestionMethod": "UTILITY_WEBSITE_ACCESS",
"discoveredAt": "2024-12-12T22:33:14.975Z",
"dueDate": "2024-12-12",
"id": "string",
"invoiceNumber": "string",
"normalizedSummaryAccountNumber": "string",
"outstandingBalance": 0,
"periodEndDate": "2024-12-12",
"periodStartDate": "2024-12-12",
"provider": {
"country": "string",
"id": "string",
"isIntervalDataSupported": true,
"isRealTimeCredentialValidationSupported": true,
"name": "string"
},
"statementDate": "2024-12-12",
"summaryAccountNumber": "string",
"totalCharges": 0,
"type": "BILL"
}
]
}
4. Filtering Meters
One distinction between statement data and interval data is that Arcadia automatically activates the statement product by default, but intervals are opt-in for each meter. That means you must choose which meters you want interval data for and then call an API endpoint to activate the intervals product explicitly.
So we first need to find the Meters to activate. Fetch all the meters associated with the correlationId
you created. Meters are created when we find them on Statements so, like statements, these meter entities may take a few minutes to be created after the credential is submitted. It's also worth noting that most residential buildings have a single account with a single meter but some have multiple (and most commercial buildings have multiple).
Again, you'll need to plug in your own accessToken
and correlationId
to call the List Meters endpoint:
curl --request GET \
--url https://api.arcadia.com/plug/meters?search=correlationIds%3D%3D{correlationId}&page=0&size=20 \
--header 'Arcadia-Version: 2024-02-21' \
--header 'accept: application/json' \
--header 'authorization: Bearer {accessToken}'
You'll get back a response with one or more meter entities:
{
"page": {
"size": 20,
"totalElements": 1,
"totalPages": 1,
"number": 0
},
"meters": [
{
"accounts": [
{
"accountNumber": "111111111",
"id": "act_1efb8ddd-52b9-d118-bf5e-867dc2fc6afd"
}
],
"bulbType": null,
"correlationIds": [
{correlationId},
],
"createdAt": "2024-12-12T23:07:18.000+00:00",
"createdBy": "system",
"currentTariff": null,
"customData": {},
"earliestIntervalAt": null,
"generalDescriptionAsPrinted": null,
"id": "mtr_11111111-1111-1111-bf5e-867dc2fc6afd",
"isIntervalsProductActive": false,
"isIntervalsThirdPartyPortalMeter": false,
"isLocationRecommendationIgnored": false,
"isStandalone": false,
"lastModifiedAt": "2024-12-12T23:07:18.000+00:00",
"lastModifiedBy": "system",
"latestIntervalAt": null,
"latestStatementDate": null,
"localTimezone": null,
"meterConstantMultiplier": null,
"meterNumber": "111111111",
"nextExpectedPostDate": null,
"normalizedMeterNumber": "11111111",
"normalizedPointOfDeliveryNumber": "11111111",
"pipeType": null,
"pointOfDeliveryNumber": "11111111",
"previousMeterConstantMultiplier": null,
"previousMeterNumber": null,
"provider": {
"country": null,
"id": "prv_36e14462-ea0a-11e0-b6bb-12313d2b6294",
"isIntervalDataSupported": true,
"isRealTimeCredentialValidationSupported": false,
"name": "Pacific Gas and Electric (PG&E)"
},
"serviceAddress": {
"addressType": "FULL",
"city": "ArcadiaVille",
"country": null,
"fullAddress": "Jane Doe, 123 Happy St, ArcadiaVille CA 96401",
"postalCode": "93619-5349",
"recipient": null,
"state": "CA",
"streetLine1": "123 Happy St",
"streetLine2": null
},
"serviceType": "electric",
"serviceTypeClassification": "CORE",
"site": null,
"status": "INTERVALS_NOT_REQUESTED",
"statusDetail": "INTERVALS_EXTRACTION_NOT_REQUESTED"
}
]
}
Notice that the Meter.isIntervalsProductActive
is False
and the Meter.status
is "INTERVALS_NOT_REQUESTED"
. That's because we haven't yet activated the interval data product which we'll do in the next step.
5. Activating Meters
In order to activate a meter, make a PATCH call to the Meter endpoint and set Meter.isIntervalsProductActive
to true
.
For this API call, you'll need the Meter.id
from the previous step and, as always, your accessToken
:
curl --request PATCH \
--url https://api.arcadia.com/plug/meters/{Meter.id} \
--header 'Arcadia-Version: 2024-02-21' \
--header 'accept: application/json' \
--header 'authorization: Bearer {accessToken}' \
--header 'content-type: application/json' \
--data '
{
"isIntervalsProductActive": true
}
'
If the call is successful, you'll see an API response that indicates that Meter.status
is "INTERVALS_IN_PROGRESS"
.
{
"page": {
"size": 20,
"totalElements": 1,
"totalPages": 1,
"number": 0
},
"meters": [
{
"accounts": [
{
"accountNumber": "111111111",
"id": "act_11111111-52b9-d118-bf5e-867dc2fc6afd"
}
],
"bulbType": null,
"correlationIds": [
{correlationId}
],
"createdAt": "2024-12-12T23:07:18.000+00:00",
"createdBy": "system",
"currentTariff": null,
"customData": {},
"earliestIntervalAt": null,
"generalDescriptionAsPrinted": null,
"id": "mtr_11111111-5614-d734-bf5e-867dc2fc6afd",
"isIntervalsProductActive": true,
"isIntervalsThirdPartyPortalMeter": false,
"isLocationRecommendationIgnored": false,
"isStandalone": false,
"lastModifiedAt": "2024-12-12T23:19:18.000+00:00",
"lastModifiedBy": "Name",
"latestIntervalAt": null,
"latestStatementDate": null,
"localTimezone": null,
"meterConstantMultiplier": null,
"meterNumber": "11111111",
"nextExpectedPostDate": null,
"normalizedMeterNumber": "11111111",
"normalizedPointOfDeliveryNumber": "11111111",
"pipeType": null,
"pointOfDeliveryNumber": "1111111",
"previousMeterConstantMultiplier": null,
"previousMeterNumber": null,
"provider": {
"country": null,
"id": "prv_36e14462-ea0a-11e0-b6bb-12313d2b6294",
"isIntervalDataSupported": true,
"isRealTimeCredentialValidationSupported": false,
"name": "Pacific Gas and Electric (PG&E)"
},
"serviceAddress": {
"addressType": "FULL",
"city": "ArcadiaVille",
"country": null,
"fullAddress": "Jane Doe, 123 Happy St, ArcadiaVille CA 96401",
"postalCode": "93619-5349",
"recipient": null,
"state": "CA",
"streetLine1": "123 Happy St",
"streetLine2": null
},
"serviceType": "electric",
"serviceTypeClassification": "CORE",
"site": null,
"status": "INTERVALS_IN_PROGRESS",
"statusDetail": "INTERVALS_EXTRACTION_IN_PROGRESS"
}
]
}
6. Fetching Interval Data
After a few minutes, interval data collection should be complete. You can start polling for interval data using your accessToken
and the Meter.id
you used in the API call to activate the meter. Simply List Intervals for that Meter using the API:
curl --request GET \
--url https://api.arcadia.com/plug/intervals/meters/{Meter.id} \
--header 'Arcadia-Version: 2024-02-21' \
--header 'accept: application/json' \
--header 'authorization: Bearer {accessToken}'
If the Interval data isn't ready just yet, you'll get a HTTP 400 error that looks like this:
{
"errors": [
{
"details": {
"errorId": "err_3jhGDQGoVICPV56566Hng"
},
"message": "Interval data is not yet ready for Meter mtr_111111_1111_11111",
"param": null,
"type": "invalidParameter"
}
]
}
You can optionally poll the Get Meter endpoint using the Meter.id
and wait for the Meter.status
to equal "INTERVALS_SUCCESS"
instead. Once the data collection is complete, you can hit that same List Intervals API endpoint and your interval data will be returned:
{
"correlationIds": [
{correlationId},
],
"credentialIds": [
"crd_11111111-ca5c-dfc4-9dd3-ba7ee6dbebcb"
],
"earliestIntervalAt": "2024-05-01T07:00:00.000+00:00",
"electricMeterMultiplier": 1,
"intervalsCreatedAt": "2024-12-12T23:24:50.000+00:00",
"intervalsUpdatedAt": "2024-12-12T23:24:50.000+00:00",
"latestIntervalAt": "2024-12-11T07:00:00.000+00:00",
"localTimezone": "America/Los_Angeles",
"meterId": "mtr_11111111-5614-d734-bf5e-867dc2fc6afd",
"meterNumber": "1011195820",
"providerId": "prv_36e14462-ea0a-11e0-b6bb-12313d2b6294",
"providerName": "Pacific Gas and Electric (PG&E)",
"readType": null,
"readings": [
{
"startAt": "2024-05-01T07:00:00.000+00:00",
"endAt": "2024-05-01T08:00:00.000+00:00",
"direction": "net",
"kwh": 0.0744,
"kw": 0.0744
},
{
"startAt": "2024-05-01T07:00:00.000+00:00",
"endAt": "2024-05-01T08:00:00.000+00:00",
"direction": "import",
"kwh": 0.0744,
"kw": null
},
{
"startAt": "2024-05-01T08:00:00.000+00:00",
"endAt": "2024-05-01T09:00:00.000+00:00",
"direction": "net",
"kwh": 0.0738,
"kw": 0.0738
},
{
"startAt": "2024-05-01T08:00:00.000+00:00",
"endAt": "2024-05-01T09:00:00.000+00:00",
"direction": "import",
"kwh": 0.0738,
"kw": null
},
...
Congrats! You've successfully pulled statement and interval data out of the Arcadia platform!
Updated 2 days ago
After you complete this Quickstart, we recommend checking out the full Sandbox Guide, dive into the rich Arcadia Data Model docs, and read the more in-depth Interval Data Guide.