Connect guide
A guide on how Connect works and how to implement it
What is Connect?
Connect is the UI component that allows you to create a connection to your users' utility account data in your application. A utility provider connection made through the Connect workflow is required for your app to use the functionality of Arc's Plug, Bundle, and Spark (Connect is NOT needed for Genability Signal and Switch).
Connect does an authentication handshake with users' utility providers, using account credentials provided by the user.
How Do I Use Connect?
There are two options for directing your customers to Connect depending on what works best for your application and desired user experience: Connect URL and Connect React.
Connect URL is an easy, lightweight way to get your users submitting credentials quickly. You will use the API to generate a Connect URL which you can send directly to the your user. When the user opens the URL, it will present Connect on a standalone webpage. After your user submits their credentials, they can close the browser tab.
Connect React is great for embedding the component directly into your own React application. This option allows for a more native feel to the user experience because the Connect component lives directly within your application rather than opening in a separate webpage. It does require more development effort than the Connect URL method.
How it works
You will present Connect to your users when you would like to create or update a connection to that user's utility provider. This connection is the foundation of all of Arc's functionality and is required to use Plug, Bundle, or Spark for your users.
When to present Connect
Your app should present Connect to your users in two different cases:
- When you'd like to create a connection to one of your user's utility providers (either for the first time, or subsequent times if they have accounts with more than one utility provider)
- When you need to update an existing connection to a user's utility provider (typically done for users that have changed their utility account password)
Connect URL
What do I need to do to get ready for Connect URL
- Get access to Arc's developer dashboard and get your sandbox API keys
- Read the Connect URL endpoint documentation
Fetching a Connect URL to Create a Utility Credential
There are a few steps you need to fetch a Connect URL create a Utility Credential:
-
Retrieve the unique ID for your user from your system. If you don't already have one, it's recommended you provide and persist a UUID. This ID will be used in Connect and the other Arc endpoints as the
client_user_id
parameter. -
Send a request to the the Connect URL endpoint by supplying the Access Token in the header of the request and providing the unique ID for the user as the
client_user_id
body parameter. -
A URL will be returned which you can load in a new tab, email to the user, or deliver to the user in whichever means makes the most sense for your application. The URL will be valid for 5 days.
Fetching a Connect URL to Update a Utility Credential
There are a few steps you need to fetch a Connect URL to update a Utility Credential:
-
Retrieve the
utility_credential_id
for the user whose credentials are being updated either by fetching it from your applications database or by querying the List Utility Credential API and providing the user'sclient_user_id
as a search filter. -
Send a request to the the Connect URL endpoint by supplying the Access Token in the header of the request and providing the
utility_credential_id
as a body parameter. -
A URL will be returned which you can load in a new tab, email to the user, or deliver to the user in whichever means makes the most sense for your application. The URL will be valid for 5 days.
Listening for Connect URL Events
You may want your application to be notified when a customer finishes going through the Connect workflow so that you can direct the user to the next part of your experience. In order to do so, you'll need to you will need to pass the parent window's origin to the iframe src
url. Here is a minimal reproduction:
<!DOCTYPE html>
<html>
<head>
<title>Connect</title>
</head>
<body>
<h1>Connect</h1>
<p>Connect your utility account to Arcadia Power</p>
<iframe id="myIframe"
src='' width="100%"
height="900px"></iframe>
<script>
window.addEventListener('message', function (e) {
console.log('message', e)
})
const iframe = document.getElementById('myIframe')
iframe.src = `{ENTER_YOUR_CONNECT_URL_HERE}`
</script>
</body>
</html>
When the end user submits credentials you will get a MessageEvent
posted to the window with contents in the form of:
{
payload: { utilityCredentialId: 12345678 },
type: "onCredentialsSubmitted"
}
Connect React
What do I need to do to get ready for Connect React?
- Get access to Arc's developer dashboard, where you'll be able to access our API documentation, our quickstart guide, and get your sandbox API keys.
- Walkthrough the Arc demo, which includes Connect.
- View Connect's npm package.
Creating a connection to a user's utility provider
When you would like to use Arc features for one of your users's utility accounts, your app must make a connection to that account using Arc's Connect component.
Arc can start getting your user's data as soon as they input the needed authentication information (typically a utility username and password) through the Connect workflow.
Getting a Connect Token to initialize Connect
A session of Connect is initialized with a Connect Token, which scopes the session to a specific user when creating new utility provider connection. Below are the steps to create that token.
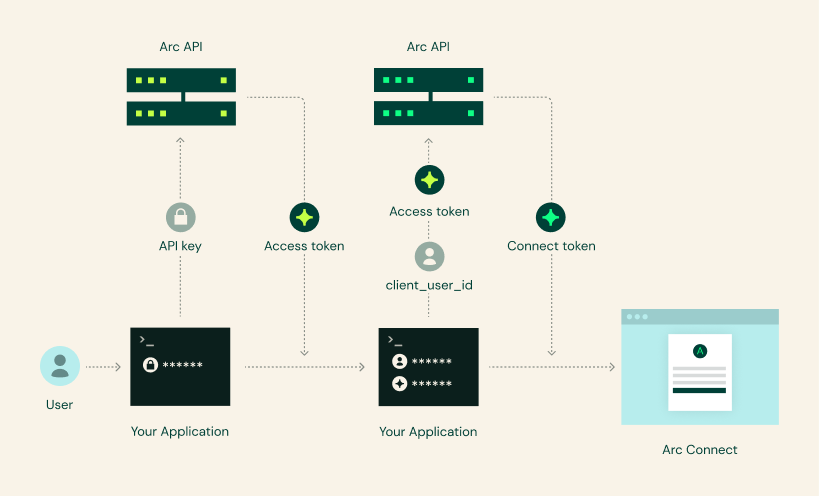
-
Retrieve the unique ID for your user from your system. If you don't already have one, it's recommended you provide and persist a UUID. This ID will be used in Connect and the other Arc endpoints as the client_user_id parameter.
-
Obtain a Connect token using the client_user_id and the access token in the authorization header. In this use case the value of the utility_credential_id parameter, which is used when the user changes their utility provider credentials, must be left empty.
-
Initialize the Connect React component with the Connect token. With the Connect token, the Connect session is scoped to the user using your app, and all accounts at the utility provider connected to in the session will be associated with the user's client_user_id.
-
Connect prompts the user to enter the data needed to authenticate with their utility provider:
- First, the user consents to allow your app (and Arc) to access their utility data. The consent includes detail on which data is obtained and a link to detailed terms and conditions.
- Next, they indicate their utility provider by entering their zip code and accepting the pre-populated option or selecting from the drop-down list.
- Finally, they supply their login credentials for the chosen utility provider. 2FA authentication is supported for a number of utilities that have implemented it.
-
Arc sends along the user's credentials to the utility provider for authentication.
-
Arc verifies the credentials with the utility provider and Connect displays the results to the user. In addition, Arc sends a webhook message with the results of the authentication. You can also poll the API. The possible authentication statuses possible are: verified, rejected, timeout, error, and pending. If pending, the result will be sent via a webhook message when ready.
At this point, Connect will return the newly created utility_credential_id to the hosting front-end application. You can use this information to poll for updates to the Utility Credential, or just listen for any changes via a webhook message.
Updating a user's utility connection
Arc regularly checks the user's utility provider's records for new statements. If the user changes their password (or other credential information) with the utility provider, the next lookup by Arc will fail.
If this happens, Arc will notify your systems via a webhook message called utility_credential_revoked. This webhook message contains the utility_credential_id mentioned in the previous section.
The next time your user uses your app, the app should prompt the user to update their utility credential information by:
- Requesting an Auth token
- Requesting a Connect token with the utility_credential_id as a parameter instead of the client_user_id (The utility_credential_id represents the specific connection between your user and the utility you are trying to update connection for)
- Initializing the Connect component with the Connect token. Because utility_credential_id was used to generate a Connect Token instead of client_user_id, the session of Connect with initialize in 'update mode'.
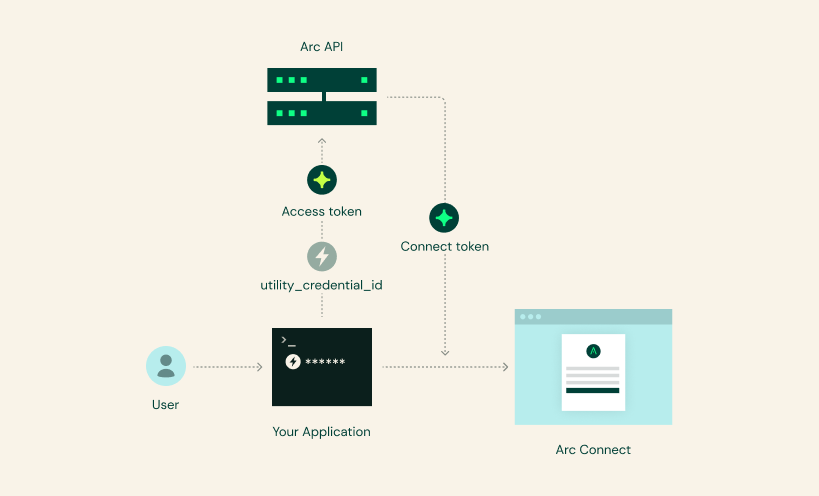
At that point the UI will prompt the user to enter their new utility provider credentials in an effort to update the broken connection. The zip code and utility selection steps of the Connect workflow will be skipped, as they are already known for the specific utility_credential_id.
Should authentication with the new credentials succeed, Arc will resume its regular statement checks and collection.
For more information...
- Try the Arc Demo to see Connect React in action
- Read the Connect URL endpoint documentation
- Read the Connect React documentation
- Read the rest of the API documentation
Updated 9 months ago